The goal was to add flexbox styles to this layout:
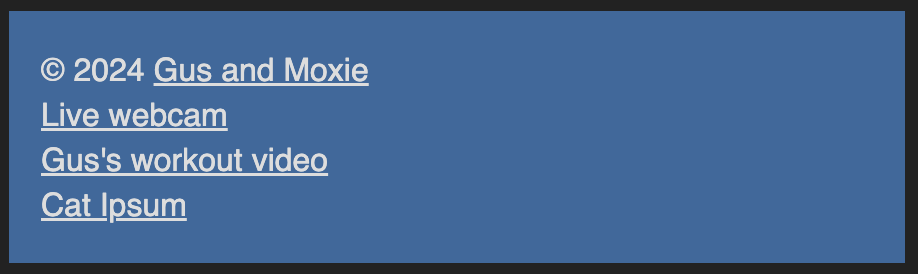
to look like this for viewport widths of 450px and wider:
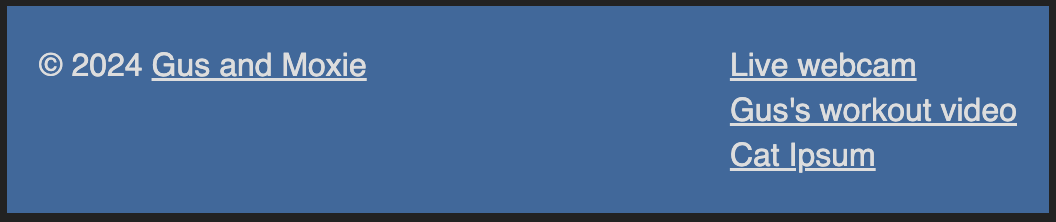
and like this for viewport widths of less than 450px:
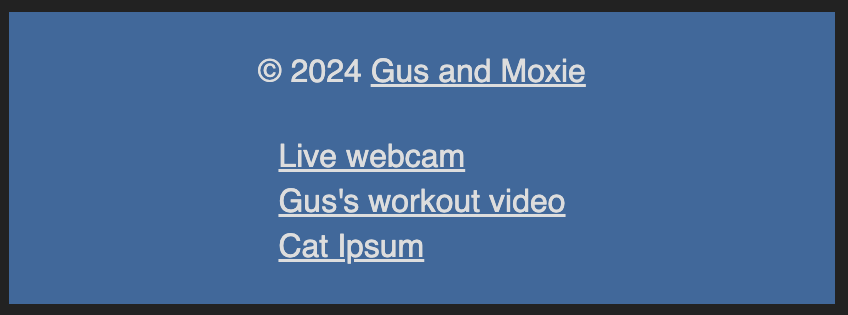
Solution
.footer {
width: 100%;
background-color: #33699e;
padding: 1rem;
box-sizing: border-box;
/* add flex styling here */
display: flex;
flex-direction: column;
align-items: center;
gap: 1rem;
}
@media (min-width: 450px) {
.footer {
/* more flex styling here */
flex-direction: row;
justify-content: space-between;
align-items: flex-start;
}
}
Explanation
The @media
query takes care of the shift from viewports less than 450px wide to viewports 450px wide and up.
In the spirit of “mobile first,” let’s start with the smaller viewport.
The HTML has taken care of grouping the links into their own div
, so that the flex container (.footer
) has only two elements: The copyright and the links.
These are vertically stacked in the narrow viewport view, so this calls for a flex-direction
of column
:
.footer {
width: 100%;
background-color: #33699e;
padding: 1rem;
box-sizing: border-box;
/* add flex styling here */
display: flex;
flex-direction: column;
}
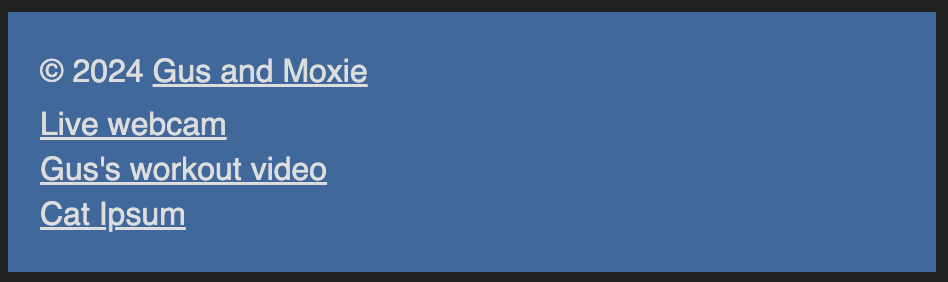
The items need to be cenetered around the primary axis (vertical, in the case of flex-direction: column
), and there’s a bit of a gap in the mock-up, too.
.footer {
width: 100%;
background-color: #33699e;
padding: 1rem;
box-sizing: border-box;
/* add flex styling here */
display: flex;
flex-direction: column;
align-items: center;
gap: 1rem;
}
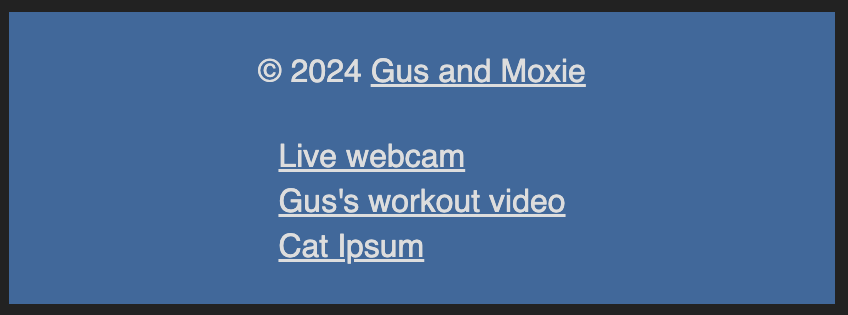
All right, now for the wider view. Here, the two elements are arranged horizontally, so the flex-direction
shifts to row
.
@media (min-width: 450px) {
.footer {
/* more flex styling here */
flex-direction: row;
}
}
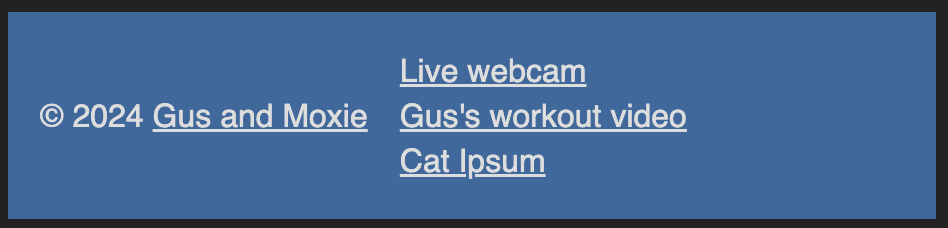
The items need to be justified along the main axis (now horizontal), putting as much space between them as possible.
@media (min-width: 450px) {
.footer {
/* more flex styling here */
flex-direction: row;
justify-content: space-between;
}
}
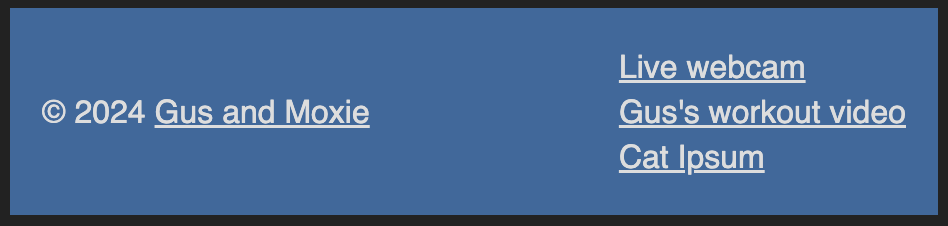
And, finally, the items need to be aligned at the top vertically, which is the secondary axis for flex-direction: row
. Since align
controls the secondary axis, we’ll use align-items
for this.
@media (min-width: 450px) {
.footer {
/* more flex styling here */
flex-direction: row;
justify-content: space-between;
align-items: flex-start;
}
}
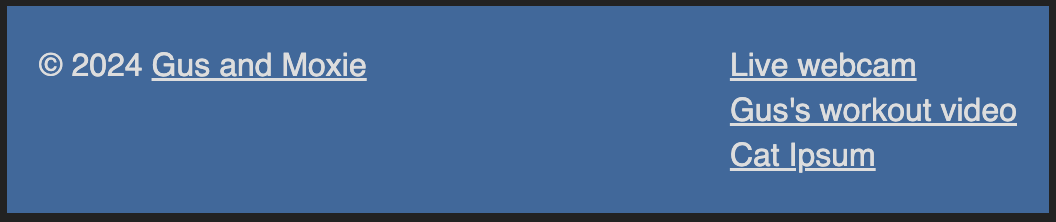
Resources
- Josh W. Comeau’s blog post, An Interactive Guide to Flexbox – great for understanding what flexbox is all about.
- A Complete Guide To Flexbox from CSS Tricks – where I go when I need quick visual reference for which flexbox properties and values I want.
- Beginner’s Guide to Media Queries from MDN